- 作者:老汪软件技巧
- 发表时间:2024-11-25 21:30
- 浏览量:
目录
Babylon.js 是一个强大的3D游戏引擎,它提供了创建3D场景所需的基础元素:包括场景、相机、光源、3D对象、基本几何体、材质、纹理、动画、物理引擎:
场景
场景是3D世界的核心,它包含了所有3D对象、相机、光源和其他元素。创建一个场景的代码如下:
// 引入Babylon.js库
import * as BABYLON from 'babylonjs';
// 创建引擎
const engine = new BABYLON.Engine(document.getElementById("renderCanvas"), true);
// 创建场景
const scene = new BABYLON.Scene(engine);
相机
相机是观察3D世界的视角,Babylon.js提供了多种类型的相机,如ArcRotateCamera、FreeCamera等。下面是一个简单的ArcRotateCamera(圆周旋转相机)的例子:
// 创建相机
const camera = new BABYLON.ArcRotateCamera("camera1",
1, // alpha(绕Y轴旋转角度)
1, // beta(绕X轴旋转角度)
10, // radius(相机距离目标点的距离)
new BABYLON.Vector3(0, 0, 0), // target(相机的目标点)
scene); // 指定场景
// 设置相机位置
camera.setPosition(new BABYLON.Vector3(0, 5, -20));
// 将相机设置为场景的默认相机
scene.activeCamera = camera;
光源
光源是给场景添加阴影和立体感的关键。Babylon.js支持多种类型的光源,如点光源、聚光灯、平行光等。
点光源(PointLight)
// 创建点光源
const pointLight = new BABYLON.PointLight("pointLight",
new BABYLON.Vector3(0, 10, 0), // 光源位置
scene); // 指定场景
// 可选:设置光源颜色和强度
pointLight.color = new BABYLON.Color3(1, 1, 1); // 白色
pointLight.intensity = 1; // 强度
聚光灯(SpotLight)
// 创建聚光灯
const spotLight = new BABYLON.SpotLight("spotLight",
new BABYLON.Vector3(0, 10, 0), // 光源位置
new BABYLON.Vector3(0, -1, 0), // 光源方向
scene); // 指定场景
// 可选:设置光源颜色、角度和强度
spotLight.color = new BABYLON.Color3(1, 1, 1); // 白色
spotLight.angle = Math.PI / 4; // 光束角度
spotLight.intensity = 1; // 强度
平行光(DirectionalLight)
// 创建平行光
const directionalLight = new BABYLON.DirectionalLight("dirLight",
new BABYLON.Vector3(-1, -1, -1), // 光源方向
scene); // 指定场景
// 可选:设置光源颜色和强度
directionalLight.color = new BABYLON.Color3(1, 1, 1); // 白色
directionalLight.intensity = 1; // 强度
3D对象
建3D对象的基本步骤包括:
创建几何体实例。创建材质实例。创建Mesh(网格)对象,将几何体和材质结合。将Mesh添加到场景中。
例如,创建一个红色的球体:
// 创建球体几何体
const sphereGeometry = new BABYLON.Sphere("sphere", 10, 20, 20); // 半径、细分水平、细分垂直
// 创建红色材质
const redMaterial = new BABYLON.StandardMaterial("redMat", scene);
redMaterial.diffuseColor = new BABYLON.Color3(1, 0, 0); // 红色
// 创建球体网格
const sphereMesh = new BABYLON.Mesh.CreateFromGeometry("sphereMesh", sphereGeometry, scene);
sphereMesh.material = redMaterial;
// 将球体添加到场景
scene.registerBeforeRender(() => {
sphereMesh.position.y += 0.1; // 上升动画
});
基本几何体Box (立方体)
// 创建立方体几何体
const boxSize = new BABYLON.Vector3(2, 2, 2); // 宽、高、深
const boxGeometry = BABYLON.BoxBuilder.CreateBox("box", { size: boxSize }, scene);
Sphere (球体)
// 创建球体几何体
const sphereRadius = 3;
const sphereSegment = 32; // 水平细分
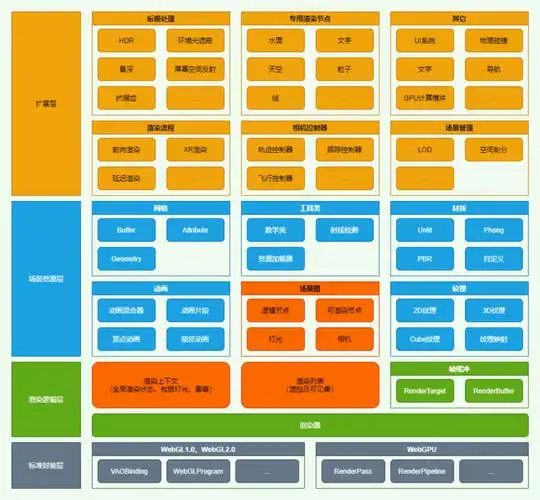
const sphereDiameter = 16; // 垂直细分
const sphereGeometry = BABYLON.SphereBuilder.CreateSphere("sphere", { diameter: sphereRadius, segments: sphereSegment, diameterSegments: sphereDiameter }, scene);
Cylinder (圆柱体)
// 创建圆柱体几何体
const cylinderHeight = 5;
const cylinderDiameterTop = 2;
const cylinderDiameterBottom = 2;
const cylinderTessellation = 32; // 分段数
const cylinderGeometry = BABYLON.CylinderBuilder.CreateCylinder("cylinder", { height: cylinderHeight, diameterTop: cylinderDiameterTop, diameterBottom: cylinderDiameterBottom, tessellation: cylinderTessellation }, scene);
材质
材质定义了3D对象的表面外观,Babylon.js提供了多种材质类型,如StandardMaterial、PBRMaterial等。
Standard Material (标准材质)
// 创建标准材质
const standardMaterial = new BABYLON.StandardMaterial("standardMat", scene);
// 设置颜色
standardMaterial.diffuseColor = new BABYLON.Color3(1, 0, 0); // 红色
standardMaterial.specularColor = new BABYLON.Color3(1, 1, 1); // 反射颜色
standardMaterial.emissiveColor = new BABYLON.Color3(0, 0, 0); // 发光颜色
// 应用材质到几何体
boxGeometry.material = standardMaterial;
Texture (纹理材质)
在标准材质上应用纹理:
// 加载纹理
BABYLON.SceneLoader.ImportMesh("", "./", "texture.jpg", scene, function (newMeshes) {
// 假设只有一个纹理被加载
const texture = newMeshes[0].material.diffuseTexture;
// 应用到标准材质
standardMaterial.diffuseTexture = texture;
});
PBR Material (基于物理的渲染材质)
PBR材质提供了更真实的光照效果,需要设置金属度、粗糙度等参数:
// 创建PBR材质
const pbrMaterial = new BABYLON.PBRMaterial("pbrMat", scene);
// 设置基础颜色和纹理
pbrMaterial.albedoColor = new BABYLON.Color3(1, 1, 1);
pbrMaterial.albedoTexture = new BABYLON.Texture("path/to/albedoTexture.jpg", scene);
// 设置金属度和粗糙度
pbrMaterial.metallic = 0.5;
pbrMaterial.roughness = 0.5;
// 应用到几何体
sphereGeometry.material = pbrMaterial;
纹理
纹理可以给3D对象添加细节和真实感。以下是如何给对象添加纹理的例子:
// 加载纹理
const texture = new BABYLON.Texture("path/to/texture.jpg", scene);
// 将纹理应用到材质
redMaterial.diffuseTexture = texture;
动画
Babylon.js提供了强大的动画系统,可以对3D对象的属性进行动画化。以下是一个简单的旋转动画:
// 创建旋转动画
const rotateAnim = new BABYLON.Animation("rotate", "rotation.y", 60, BABYLON.Animation.ANIMATIONTYPE_FLOAT, BABYLON.Animation.ANIMATIONLOOPMODE_CYCLE);
const keys = [
{ frame: 0, value: 0 },
{ frame: 100, value: Math.PI * 2 }
];
rotateAnim.setKeys(keys);
// 将动画添加到球体
sphereMesh.animations.push(rotateAnim);
// 开始播放动画
BABYLON.Animation.StartNew(sphereMesh, 0, "rotate");
物理引擎
Babylon.js集成了Cannon.js和Oimo.js物理引擎,可以实现3D物体的碰撞检测和物理模拟。以下是一个简单的物理初始化和添加刚体的例子:
// 初始化物理引擎
const physicsEngine = new BABYLON.CannonJSPlugin();
scene.enablePhysics(null, physicsEngine);
// 将球体转换为刚体
sphereMesh.physicsImpostor = new BABYLON.PhysicsImpostor(sphereMesh, BABYLON.PhysicsImpostor.SphereImpostor, { mass: 1 }, scene);
// 添加地面作为静态刚体
const ground = BABYLON.Mesh.CreatePlane("ground", 100, scene);
ground.rotation.x = -Math.PI / 2; // 使地面水平
ground.physicsImpostor = new BABYLON.PhysicsImpostor(ground, BABYLON.PhysicsImpostor.PlaneImpostor, { mass: 0 }, scene);